HTML Form Validation Using JavaScript
We are going to setup a form validation using javaScript. First, We will see the very basic concept and then create and develop a complete structure with a simple HTML contact form with our form validation using JavaScript along the way
You can use this JavaScript form validation code wherever you want like Blogger, WordPress, GitHub pages and any other platforms using HTML or PHP custom forms
Basic Source Code Form Validation Using JavaScript
source codes given below are just for understanding how it works. After this, we are going to implement this source code in a sample contact form to make a complete form with validation using javascript.
HTML elements being handled in form validation process:
4 + 2 = <input id="answerInput" type="number" value=""> <button onclick="validateForm();">Verify</button> <input type="submit" id="submitButton" value="Submit">
Javascript code to handle the form validation process:
disableSubmit(); function disableSubmit() { document.getElementById("submitButton").disabled = true; } function validateForm() { var answer = document.getElementById("answerInput"); var submit = document.getElementById("submitButton"); if (answer.value === "6") { submit.disabled = false; } else { submit.disabled = true; } }
How It Works?
HTML Part
- In the HTML section, we have defined three elements. First one is
input[type=number]
with id"answerInput"
- Second one
<button>
with anonclick="validateForm();"
function - Finally, the third one
input[type=submit]
with id"submitButton"
- The above three elements form the form validation part and submit button
- Before all these elements, we have written the innerHTML
4 + 2 =
- These are the visible HTML elements form elements where we have to input the correct answer to validate the and pass the human verification
JavaScript Part
- We have handled two JavaScript functions:
disableSubmit()
andvalidateForm()
- In the beginning of the script, the function
disableSubmit();
is being called so that it always disable the button every time the page loads. - When the VERIFY button is clicked,
validateForm()
function is being called on where it verifies whether theanswerInput
varries the correct value or not - If the input value is correct, it changes the submit button’s
disabled
mode tofalse;
otherwise totrue;
using if else conditional statements. - We have told that
"6"
is the correct answer using the if statementif (answer.value === "6")
Using the above JavaScript Validation in a Contact Form
We are now going to implement the above JavaScript form validation code in a simple HTML contact form with a couple of CSS style to shape the form to a good look.
<!DOCTYPE html> <html> <head> <title>Contact Form With JavaScript Form Validation</title> <style type="text/css"> input[type=text], input[type=number], input[type=email], textarea { width: 100%; max-width: 500px; margin-bottom: 10px; outline: none; padding: 6px 10px; border-radius: 3px; box-sizing: border-box; border: 2px solid #ccc; -webkit-transition: 0.5s; transition: 0.5s; } .contact-form { padding: 10px 20px; border: 1px solid #ccc; border-radius: 3px; background: #eee; } #answerInput { -moz-appearance:textfield; max-width:50px; text-align: center; border-radius: 3px; box-sizing: border-box; border: 2px solid #ccc; -webkit-transition: 0.5s; transition: 0.5s; outline: none; padding: 6px 10px; } input[type=submit] { background: #0073aa; color: #ffffff; text-align: center; border:0px; border-radius: 3px; box-sizing: border-box; -webkit-transition: 0.5s; transition: 0.5s; outline: none; padding: 6px 25px; margin-bottom: 10px; margin-top: 10px; cursor:pointer; } .verify-button { background: #d14836; color: #ffffff; text-align: center; border-radius: 3px; box-sizing: border-box; -webkit-transition: 0.5s; transition: 0.5s; outline: none; padding: 6px 25px; cursor: pointer; } </style> </head> <body> <!--Contact Form Starts Here--> <div class="contact-form"> <h1>Contact Form</h1> <form action=""> Name:<br/> <input type="text" name="name"><br/> Email:<br/> <input type="email" name="email"><br/> Message:<br/> <textarea name="message"></textarea><br/> <!--Form Validation Starts--> <div class="validation"> 4 + 2 = <input id="answerInput" type="number" value=""> <span class="verify-button" onclick="validateForm();">Verify</span><br/> </div> <div id="validationMessage"></div><br/> <!--Form Validation End--> <input type="submit" id="submitButton" value="Submit"> </form> </div> <!--Contact Form End--> <script type="text/javaScript"> disableSubmit(); function disableSubmit() { document.getElementById("submitButton").disabled = true; } function validateForm() { var answer = document.getElementById("answerInput"); var submit = document.getElementById("submitButton"); var validation = document.getElementById("validation") var message = document.getElementById("validationMessage") if (answer.value === "6") { submit.disabled = false; message.innerHTML = "Verification Succeed!"; message.style.color = "green"; validation.style.border = "none"; validation.style.padding = "0px"; } else { submit.disabled = true; message.innerHTML = "Verification failed. Please try again!"; message.style.color = "Red"; validation.style.border = "solid red"; validation.style.padding = "10px"; } } </script> </body> </html>
To preview the above code how it works, just see our contact page. In our contact page, the contact form design may be different but, the form validation method works the same as explained above.
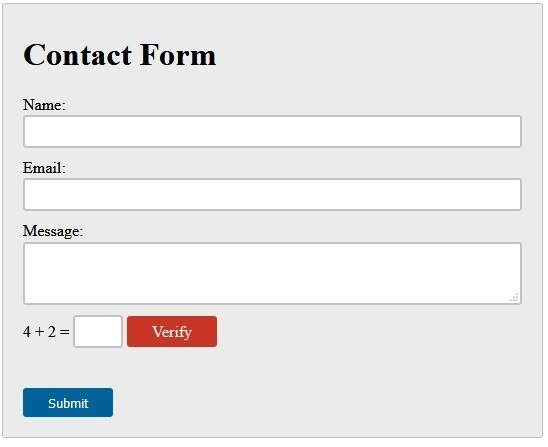
In our final complete HTML code, you may find a lot of variations from our basic validation script explained initially. The reasons for the changes are as follows:
- The above code contains a complete HTML form with validation using JavaScript with CSS style
- We have used
<span>
tag in place of VERIFY button . This is because, if we use a button orinput[type=submit]
, the form will try to submit the form and the page will get reloaded. So, to avoid this, we are using<span>
tag. - We have added some additional JavaScript statements to perform further more actions like highlighting the validation error, etc.
- We have included some CSS styles to beautify our HTML form
Just save the above code as a HTML file and open it in your browser, you will see a simple and cute HTML contact form with validation using JavaScript. If you want you modify the CSS style and change the design of the contact form as your wish.
Post a Comment
0 Comments